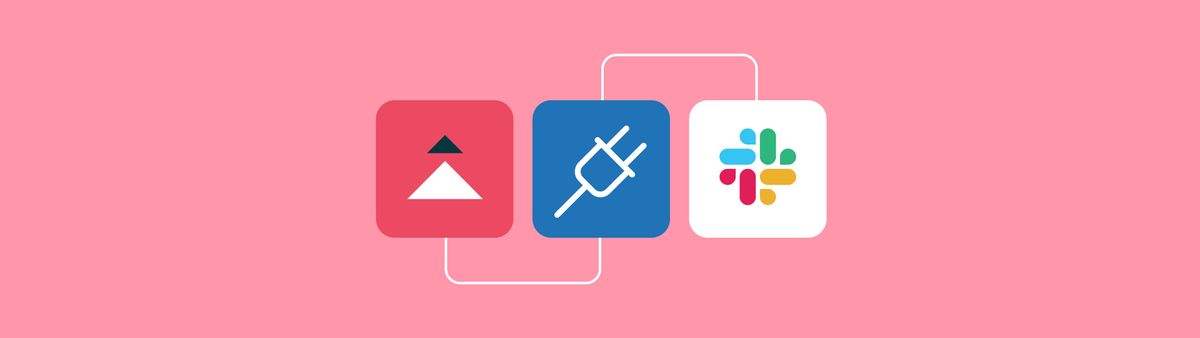
Publish new Articles to Slack via the new Webhooks for Zendesk Help Center
Learn how to publish Zendesk Guide articles to Slack by using the new Zendesk Events for Guide webhooks.
Zendesk announced webhooks for their Help Center this week. A pretty cool new expansion on the existing Zendesk Event Webhooks feature introduced last year. Building on top of the existing users, agent, groups and organisation events, you can now get notified for changes in your articles (or community).
You can get notified on the following Help Center events:
- Any help center article events
- Article published and/or unpublished
- Article subscription created
- Article vote created/changed/removed
- Article comment published/unpublished/created/changed
And for each event you get a POST request to a webhook endpoint of your choice:
{
"account_id": 10168721,
"detail": {
"brand_id": "224348602",
"id": "7005414006398"
},
"event": {
"author_id": "225859532",
"category_id": "7005437869694",
"locale": "en-us",
"section_id": "7005437870078",
"title": "James Bond Gadgets"
},
"id": "01GX4P86AC7T0AFF86QGAHTEFR",
"subject": "zen:article:7005414006398",
"time": "2023-04-03T23:11:49.571545199Z",
"type": "zen:event-type:article.published",
"zendesk_event_version": "2022-11-06"
}
What's possible now?
I used to follow all sections on my Help Centers with a dedicated end-user and then monitor that users' mailbox for changes. I wuld then use Zapier or Cloudflare Workers for Email to automate flows based on received emails to monitor and then automate based on changes in Guide.
These new webhooks allow me to tear down that Rube Goldberg Machine of an automation flow and use cleaner and more efficient code.
Examples:
- Notify your teams via Slack about article publications
- Post new articles to Twitter, Facebook or other socials via Zapier
- Notify your Marketing team so they can add it to release notes
- ...
In this article we'll build a quick example that pushes any new article to Slack to notify your internal teams.
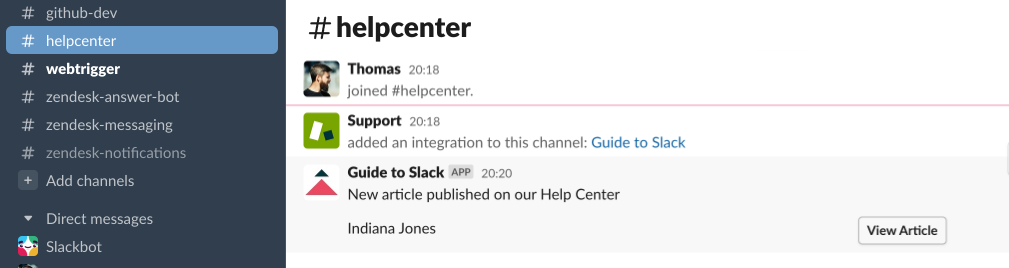
Every new subscriber motivates me to keep putting in the effort.
Thanks,
Thomas