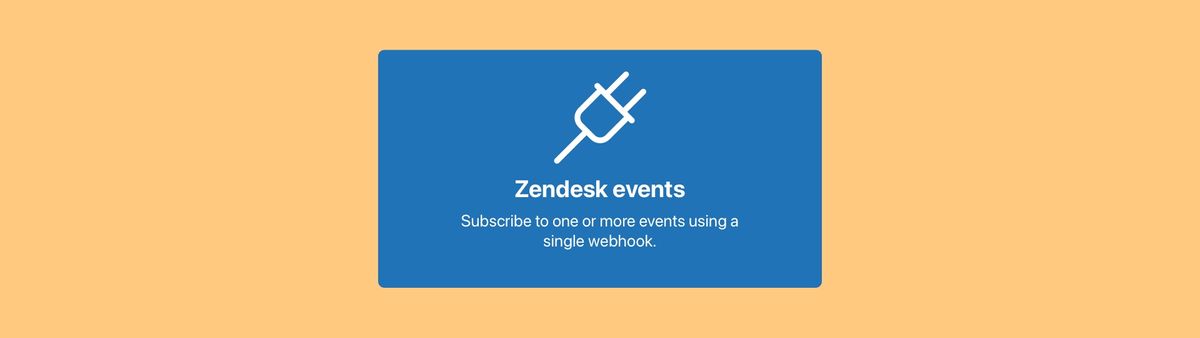
Webhooks for User and Organisation events
Zendesk recently launched an expansion on their webhooks functionality that allows you to subscribe to changes in Users and Organizations and act upon those actions. In this article we'll show how you can auto-complete agent profiles with a signature, alias and profile image upon creation.
Zendesk recently launched an expansion on their webhooks functionality that allows you to subscribe to changes in Users and Organizations and act upon those actions.
Turning theoretical functionality into a real use case can be daunting, so in this article we'll highlight one use case to show what's possible with this cool new feature.
Completing Agent Profiles
When an admin adds a new Agent to the Agent Workspace Zendesk only asks for a name and email address. But often, we need to add more data to the profile: signature, alias, verify the email address, or add a Profile Picture.
Instead of doing this manually for each agent, we can leverage the Zendesk Event Webhooks and an external script to automatically complete profiles upon creation.
In our scenario we subscribe to the Support User Created event zen:event-type:user.created
to get notified whenever a new user has been added to your instance.
We then run a script on Cloudflare Workers that will grab the incoming user ID, checks if this is an Agent, and adds a few items to their profile.
More info here