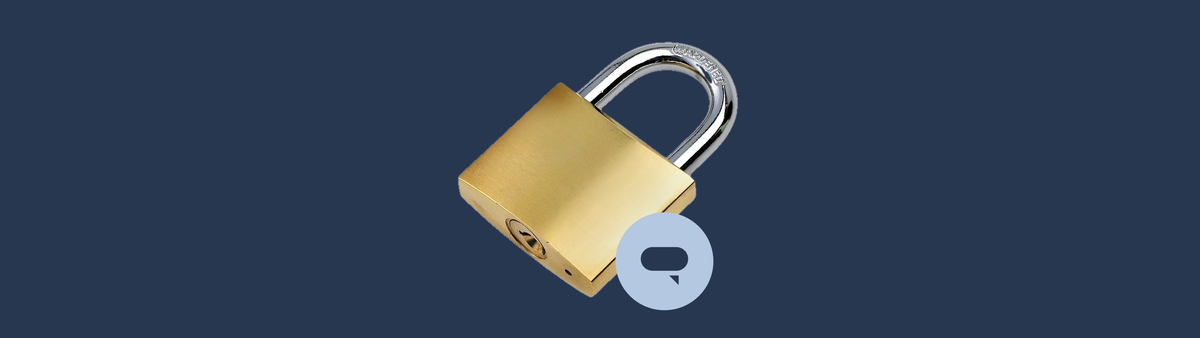
Authenticate Zendesk Messaging
Zendesk recently added the ability to authenticate users in the Zendesk Messaging Web and Mobile SDK. This article shows how to set it up with sample code.
This means Email matching is still an issue, but External IDs do work!
Zendesk recently added the ability to authenticate users in the Zendesk Messaging Web and Mobile SDK. This article shows how to set it up with sample code.
Zendesk recently added the ability to authenticate users in the Zendesk Messaging Web and Mobile SDK. This allows any website or app that has logged in users to pass that information to Zendesk so that you're sure you're talking to the right person, and removes the need for your customer to enter any credentials.
Setting it up is easy, as long as you have a working JWT endpoint. If you haven't got one, this guide will show you how to set one up using Cloudflare Workers.