➕Pre-filling custom fields in the Zendesk Bot with Messaging Metadata
This article contains three tutorials that explain how to use the new Messaging Metadata feature in Zendesk to pre-fill fields in your conversation bot flows.
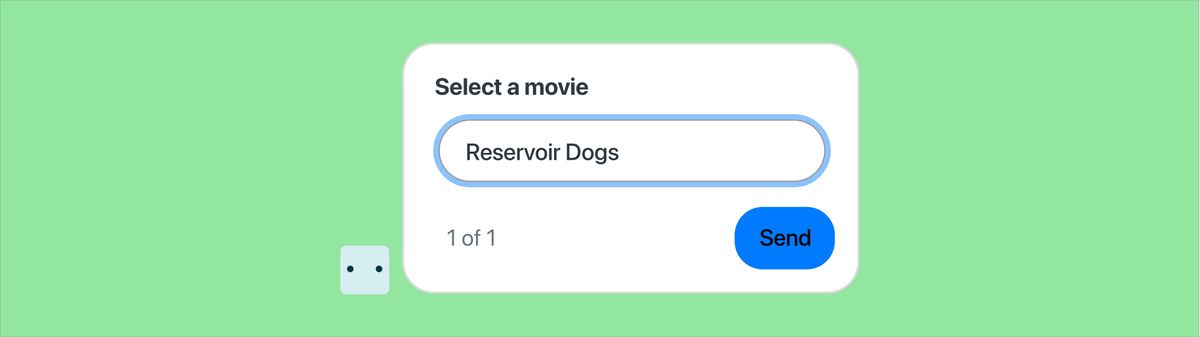
Last week's article showed how Zendesk now allows you to show restricted Help Center articles in Guide, removing one of the final missing items for feature parity between the Classic Widget and Messaging from the list.
Less than a week later they released another update to Messaging that resolves maybe the biggest open item on that list:
In short, it is now possible to fill in custom fields in the Messaging flows via a bit of code and preselecting dropdown, pre-filling text fields or checking checkboxes without user interaction.
This not only enables now flows in your Conversational Bot, but also removes manual actions that used to be done by your users: you can enter order numbers, select a venue, tag conversations with VIP, or set values you can use in API calls (retrieve an order) or branched conditions in your flows.
This is all done with one fairly simple line of code:
zE('messenger:set', 'conversationFields', [{ id: 'id', value: 'string'}]);
Let's dive in!
Pre-filling Ticket Fields
To demo this new feature I've build three demo environments:
- Movie Picker - a demo on how to pre-fill a text field and use the input to make an API call.
- Star Tours - a demo on how to choose a dropdown option and handle the choice via conditional branching.
- VIP Flow - set a VIP tag whenever a VIP customer is logged in and contacts you via the bot.
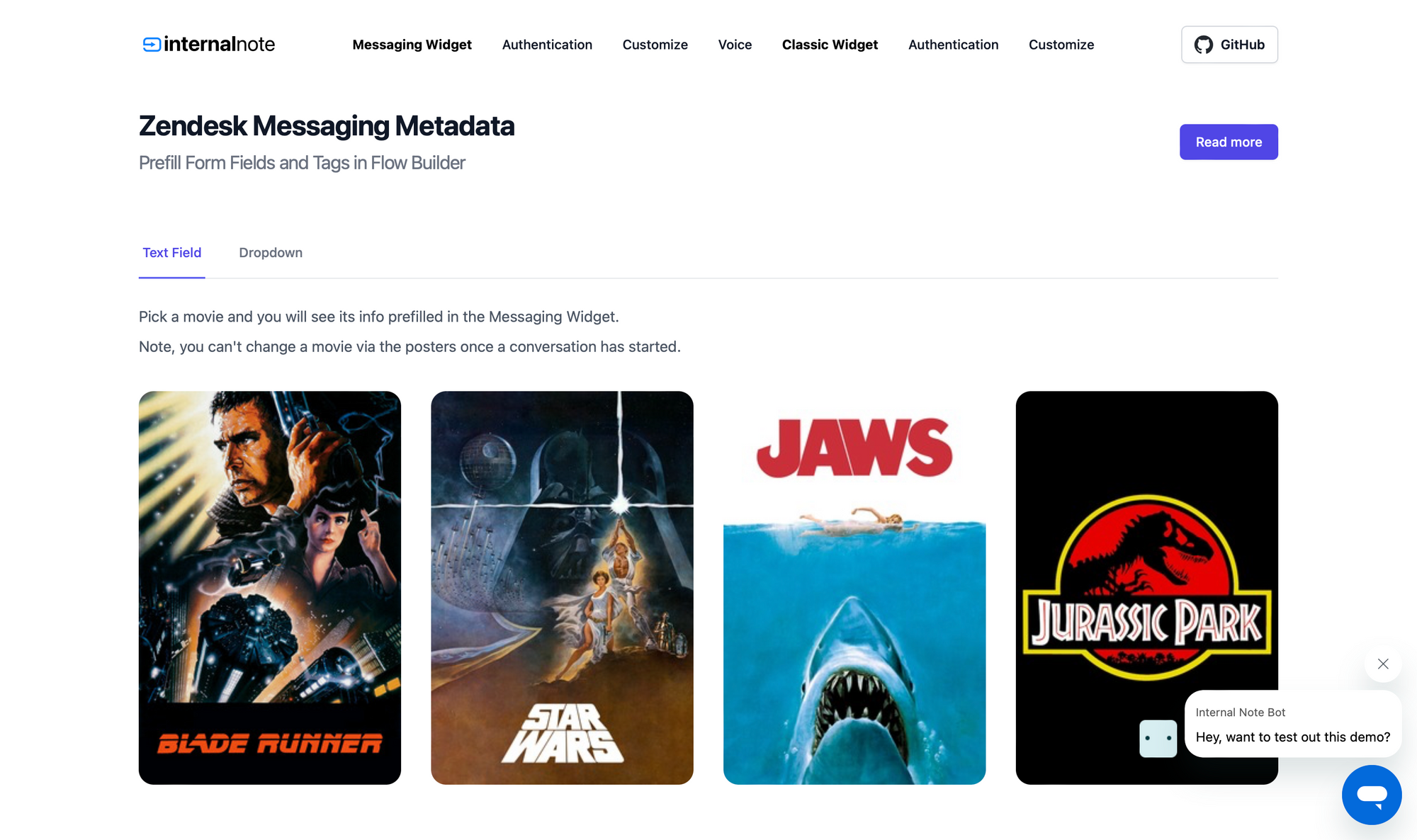
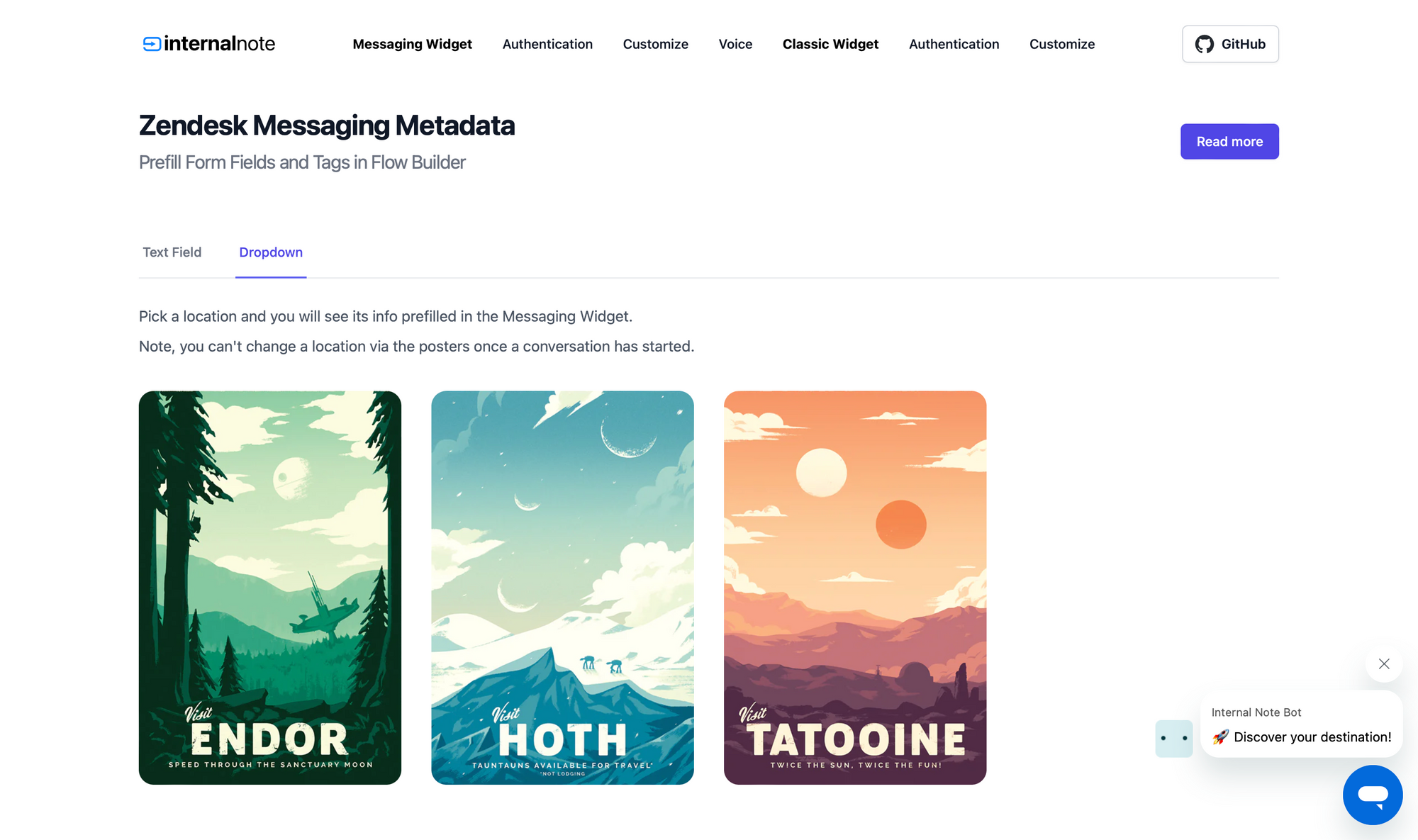